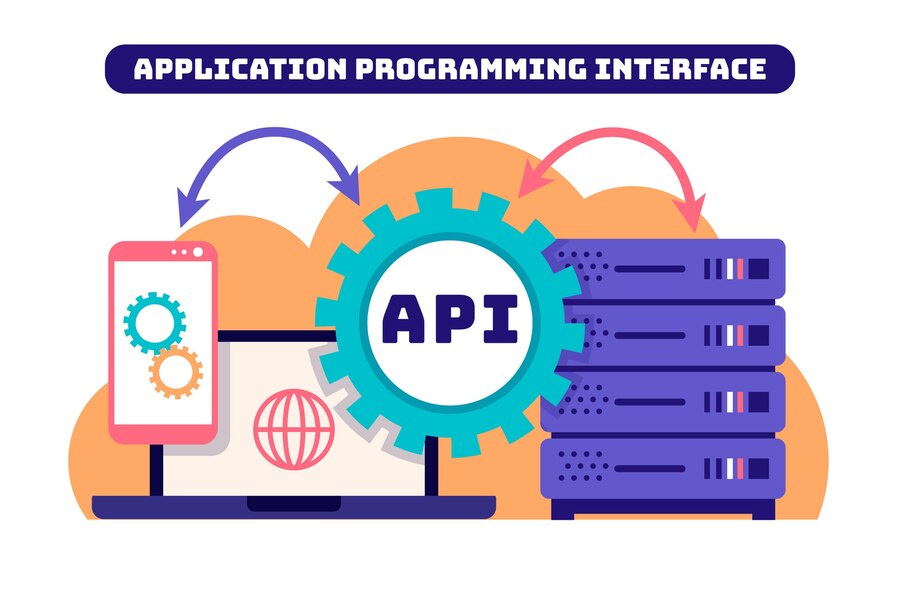
Handling Dynamic Routes in API Middleware
Handling Dynamic Routes in API Middleware Introduction: Dynamic routes are a powerful feature of Next.js, allowing you to create pages or APIs with parameters like /user/[id]. However, when using middleware to handle requests to dynamic routes, developers often face issues such as incorrect parameter extraction or unintended behavior. In this blog, we’ll explore one such problem, discuss the root cause, and provide a solution with examples. Uncaught TypeError: Cannot Read Property ‘X’ of Undefined The Problem: Imagine you’re building a user profile page with an API route /api/user/[id]. You add middleware to log user activity and restrict access based on roles. However, you notice that the middleware doesn’t correctly handle dynamic parameters like [id]. For instance: Parameters are sometimes undefined. Middleware runs even on routes where it shouldn’t. import { NextResponse } from ‘next/server’; export function middleware(req) {const { pathname } = req.nextUrl;console.log(‘Pathname:’, pathname); // Expect ‘/api/user/123’ if (pathname.startsWith(‘/api/user’)) {const userId = pathname.split(‘/’).pop();console.log(‘User ID:’, userId); // Sometimes returns undefined!} return NextResponse.next();} When you access /api/user/123, the userId may not resolve correctly, leading to errors. Understanding the Issue The issue arises because middleware in Next.js operates on the URL’s pathname, not the resolved dynamic parameters. If you rely on simple string manipulation without validating the route structure, you’ll encounter errors when the path doesn’t exactly match your expectation. The Solution: To fix this, use the nextUrl.pathname property and validate it against the dynamic route structure. Here’s an updated version: import { NextResponse } from ‘next/server’; export function middleware(req) {const { pathname } = req.nextUrl; // Check if the route matches the dynamic structureconst dynamicRoute = /^/api/user/(d+)$/;const match = pathname.match(dynamicRoute); if (match) {const userId = match[1]; // Extract the dynamic parameterconsole.log(‘User ID:’, userId); // Correctly logs ‘123’ // Perform additional checks or actionsif (parseInt(userId) > 1000) {return new NextResponse(‘User not allowed’, { status: 403 });}} return NextResponse.next();} Explanation Regex Validation: The dynamicRoute regex ensures the route matches the expected structure. Parameter Extraction: Using match[1], you extract and validate the dynamic parameter. Conditional Logic: You can now add role-based access checks or logging safely. Handling SEO Challenges with Dynamic Routes in Next.js Introduction: Dynamic routes in Next.js are a powerful feature, allowing developers to create pages or… Read More Managing WebSocket Connections in Next.js API Routes Introduction: WebSockets are essential for real-time applications like chat apps, live updates, and collaborative… Read More Fixing next/image Issues with External Domains Introduction: The next/image component is a powerful tool in Next.js for optimizing images, but developers… Read More Solving Next.js Build Errors with Custom Babel Configurations Introduction: Next.js simplifies the development process with its built-in configurations, but when you… Read More Performance Bottlenecks in API Routes with Large Payloads Performance Bottlenecks in API Routes with Large Payloads Introduction: API routes in Next.js are designed… Read More Dealing with Unexpected Re-renders in SSR Dealing with Unexpected Re-renders in SSR Introduction: React components in server-side rendered (SSR)… Read More Managing Asset Loading in Custom Server Environments Managing Asset Loading in Custom Server Environments Introduction: Custom servers in Next.js provide… Read More Handling Dynamic Routes in API Middleware Handling Dynamic Routes in API Middleware Introduction: Dynamic routes are a powerful feature of Next.js,… Read More