Fixing next/image Issues with External Domains
Introduction:
The next/image
component is a powerful tool in Next.js for optimizing images, but developers often encounter issues when trying to load images from external domains. These problems typically arise because Next.js enforces strict domain policies for security and performance reasons.
In this blog, we’ll delve into the root cause of these issues and demonstrate how to configure next/image
to handle external domains effectively.
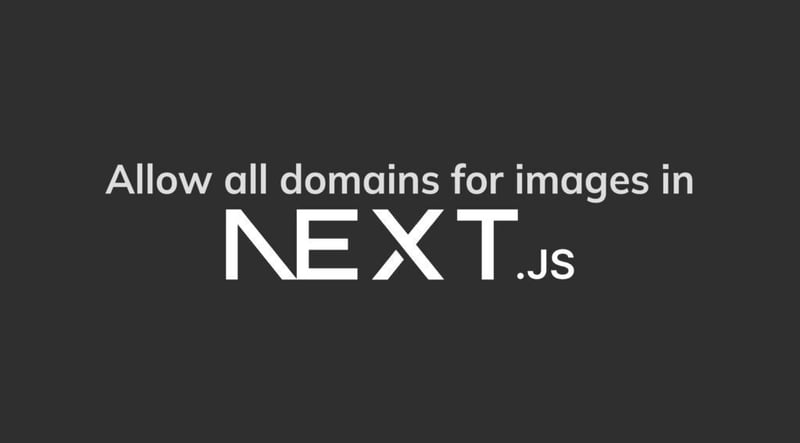
Uncaught TypeError: Cannot Read Property ‘X’ of Undefined
The Problem:
You’re building a Next.js website that relies on external image URLs, such as those from a third-party CMS or CDN. When you try to display these images using the next/image
component, they fail to load, and you see the following error in your browser console:
Error: Invalid src prop (external-domain.com/image.jpg) on `next/image`, hostname is not configured under images in next.config.js
import Image from ‘next/image’;
export default function Example() {
return (
<Image
src=”https://external-domain.com/example.jpg”
alt=”Example Image”
width={500}
height={300}
/>
);
}
Issues
- The external domain is not included in Next.js’s
next.config.js
file. - The
next/image
component enforces strict domain whitelisting for external sources.
Understanding the Issue
Understanding the Issue
Next.js requires you to explicitly list allowed image domains in the next.config.js
file for security and to optimize image loading. Without this configuration, the framework blocks external images to prevent malicious behavior or performance degradation.
The Solution:
To fix this issue, you need to configure the images.domains
property in your Next.js configuration.
Open or create a next.config.js
file in the root of your project:
module.exports = {
images: {
domains: [‘external-domain.com’, ‘another-domain.com’], // Add allowed domains
},
};
Restart your development server to apply the changes:
npm run dev
Update your component code to use the next/image
component with the external image source:
import Image from ‘next/image’;
export default function Example() {
return (
<Image
src=”https://external-domain.com/example.jpg”
alt=”Example Image”
width={500}
height={300}
/>
);
}