Handling SEO Challenges with Dynamic Routes in Next.js
Introduction:
Dynamic routes in Next.js are a powerful feature, allowing developers to create pages or APIs with dynamic parameters like /user/[id]
or /post/[slug]
. However, one of the challenges that developers face with dynamic routes is ensuring that search engines can properly index and display dynamic content.
In this blog, we’ll explore the SEO challenges posed by dynamic routes, understand why they occur, and provide a solution to make sure your dynamic pages are fully optimized for search engines.
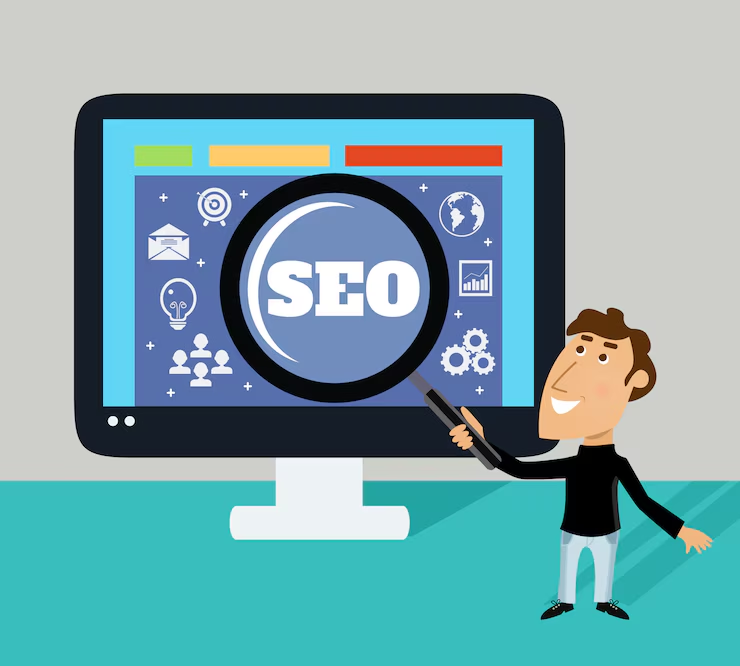
Uncaught TypeError: Cannot Read Property ‘X’ of Undefined
The Problem:
When using dynamic routes in Next.js, SEO issues arise because search engines may not be able to pre-render pages or inject necessary meta tags (such as title, description, or OG tags) for dynamic content. This leads to issues like:
- Meta Tags Not Being Pre-rendered: Search engines are unable to index dynamic pages correctly.
- Missing Dynamic Content in Search Results: Pages may appear incomplete in search results or not be indexed at all.
- Slow Crawling and Indexing: Search engines might struggle to properly crawl dynamic pages, leading to poor SEO performance.
// /pages/post/[slug].js
import { useRouter } from ‘next/router’;
export default function Post() {
const router = useRouter();
const { slug } = router.query;
return <h1>Post: {slug}</h1>;
}
Understanding the Issue
Next.js uses Static Generation (SSG) or Server-Side Rendering (SSR) for pre-rendering content. However, with dynamic routes, Next.js may not automatically generate the correct SEO-related meta tags or content for each page.
Search engines may not execute JavaScript or handle dynamic content generation effectively during crawling, meaning that dynamic pages will have incomplete metadata unless explicitly handled.
The Solution:
To fix this issue and ensure that search engines can properly index your dynamic pages, you can use getServerSideProps (SSR) to dynamically inject SEO-friendly meta tags at runtime. This allows Next.js to serve a pre-rendered page with proper metadata for each dynamic route.
Here’s how you can implement this solution:
Use getServerSideProps to Fetch Dynamic Content:
Using SSR, you can fetch data from a server-side function and render the correct meta tags for each page dynamically.Add Dynamic Meta Tags Based on Content:
Inject the appropriate meta tags, including title, description, and Open Graph tags, based on the content of the page.
// /pages/post/[slug].js
import { useRouter } from ‘next/router’;
export default function Post({ post }) {
const router = useRouter();
if (router.isFallback) {
return <div>Loading…</div>;
}
return (
<>
<head>
<title>{post.title} | My Blog</title>
<meta name=”description” content={post.description} />
<meta property=”og:title” content={post.title} />
<meta property=”og:description” content={post.description} />
<meta property=”og:image” content={post.image} />
</head>
<h1>{post.title}</h1>
<p>{post.content}</p>
</>
);
}
export async function getServerSideProps({ params }) {
const res = await fetch(`https://api.myblog.com/posts/${params.slug}`);
const post = await res.json();
return {
props: {
post,
},
};
}