Managing Asset Loading in Custom Server Environments
Introduction:
Custom servers in Next.js provide flexibility, but they can introduce challenges when resolving static assets, such as images, CSS, or JavaScript files. Developers often face issues with incorrect file paths or broken links when serving these assets.
In this blog, we’ll explore the root cause of this problem and provide a solution with proper configurations to ensure smooth asset loading.
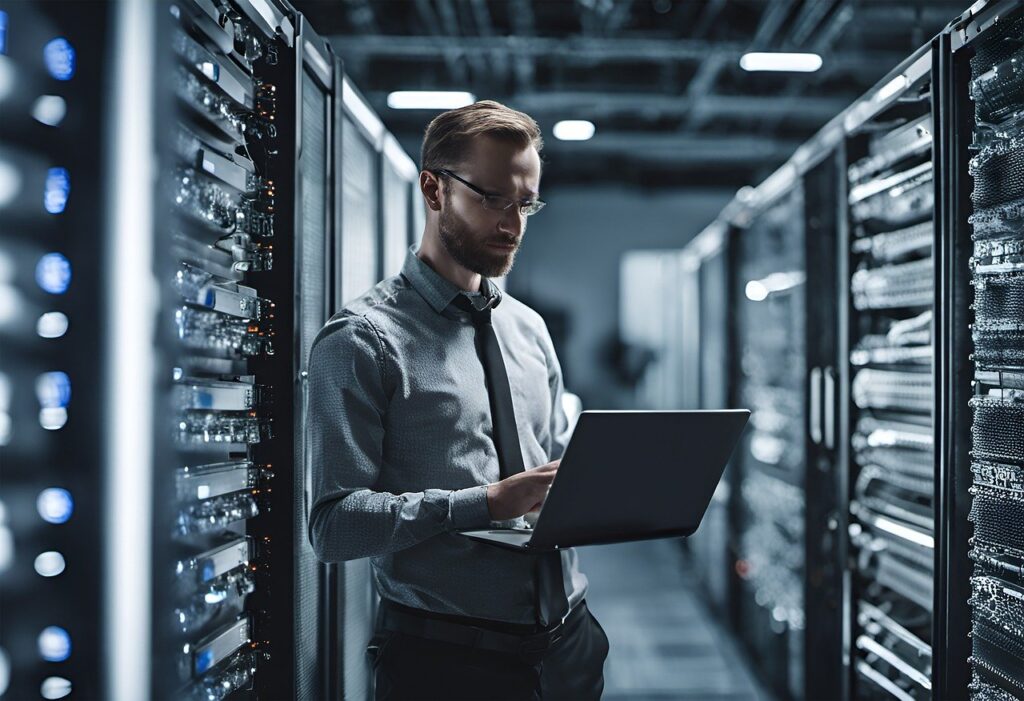
Uncaught TypeError: Cannot Read Property ‘X’ of Undefined
The Problem:
When using a custom server, static files might not resolve correctly because Next.js expects a specific structure for serving static assets, such as /public
. However, custom servers might modify the base path, causing assets to break.
const express = require(‘express’);
const next = require(‘next’);
const app = next({ dev: false });
const handle = app.getRequestHandler();
app.prepare().then(() => {
const server = express();
server.use(‘/static’, express.static(‘public’)); // Custom path
server.all(‘*’, (req, res) => handle(req, res));
server.listen(3000, () => console.log(‘Server running on http://localhost:3000’));
});
In this setup, /static/my-image.png
might not load as expected due to misconfigured paths.
Understanding the Issue
The issue arises because Next.js uses /public
as the default directory for static assets. When using a custom server, this default behavior might not align with how the server resolves file paths.
The Solution:
To ensure proper resolution, use the assetPrefix
and publicRuntimeConfig
properties in next.config.js
.
module.exports = {
assetPrefix: ‘/static’,
publicRuntimeConfig: {
staticFolder: ‘/static’,
},
};
Updated Server:
server.use(‘/static’, express.static(path.join(__dirname, ‘public’)));
Access files like this:
<img src={`${publicRuntimeConfig.staticFolder}/my-image.png`} alt=”My Image” />;
Explanation
assetPrefix
: Updates the base URL for static assets.publicRuntimeConfig
: Makes the prefix available globally in your app.- Consistent Paths: Ensures all static assets resolve correctly, even in custom setups.